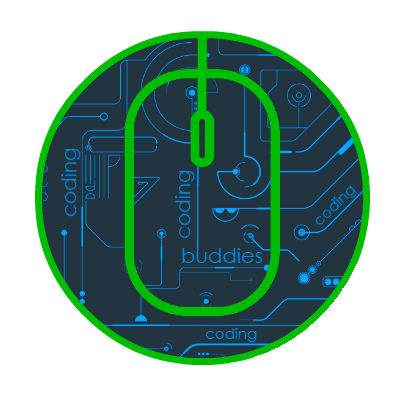
Most Recent Lesson
Printing, Variables and Input
Welcome to your first lesson!
In this lesson you will be learning the basics of input and output. You can think of programming as writing the instuctions that tells the computer what to do.
The print function is primarily used for outputting text onto the screen. Typing print "sample text"
will display the text written in the quotations onto the screen.
You can also print the value of variables by typing print variableName
. Variables are like containers where each unique variable name (i.e. "age") can hold a specific variable type, which can range from numbers, words, characters and so on. Variables can be assigned values by using an equal sign ("="). They can be changed by assigning them with new values or adding onto the existing value.
raw_input()
and input()
.
- raw_input("Optional text") - can take in any type of input, from sentences to numbers
- input("Optional text") - can take in only numbers, and unlike raw_inputs, can perform math equations with them
More Details
The main variables that are often used are strings, integers, doubles, characters, boolean.
- Strings - contain letters, words or even sentences.
- Integers - contain any whole number (including negative numbers)
- Doubles - contain decimal numbers
- Characters - contain any single character or symbol
- Boolean - are either true or false
Try It Out!
Go to Replit
- Ask for the user's name, then print it.
- Ask for a color and a number, print the number and then print the color.